libcurl c++ get post
本次博主将使用 c++
操作 libcurl
进行 get
post
摘自官网:
libcurl是一个免费且易于使用的客户端URL传输库,支持DICT、FILE、FTP、FTPS、GOPHER、GOPHERS、HTTP、HTTPS、IMAP、IMAPS、LDAP、LDAPS、MQTT、POP3、POP3S、RTMP、RTMPS、RTSP、SCP、SFTP、SMB、SMBS、SMTP、SMTPS、TELNET和TFTP。libcurl支持SSL证书、HTTP POST、HTTP PUT、FTP上载、基于HTTP表单的上载、代理、HTTP/2、HTTP/3、cookie、用户+密码身份验证(基本、摘要、NTLM、协商、Kerberos)、文件传输恢复、HTTP代理隧道等
本章节博主将使用 libcurl
完成 get
post json
post form
操作
源代码放到博文最下方了 o( ̄▽ ̄)ブ
get
服务端代码:
1 | @GetMapping("/getUser") |
c++ 代码
1 | /** |
测试调用
1 | void testGet() |
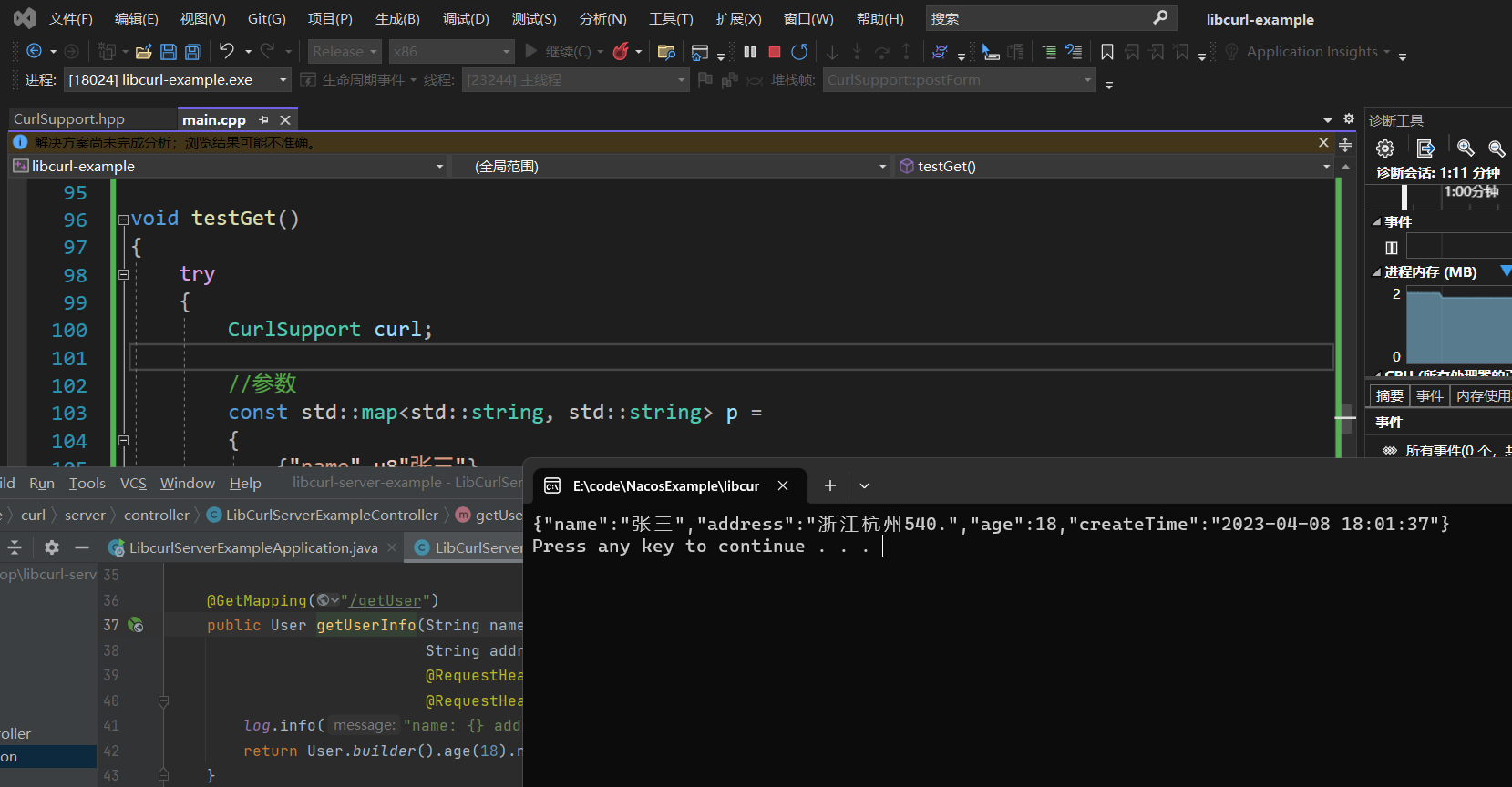
post json
服务端代码
1 | @PostMapping("/addUser") |
c++ 代码
1 | /** |
测试调用
1 | void testPostJson() |
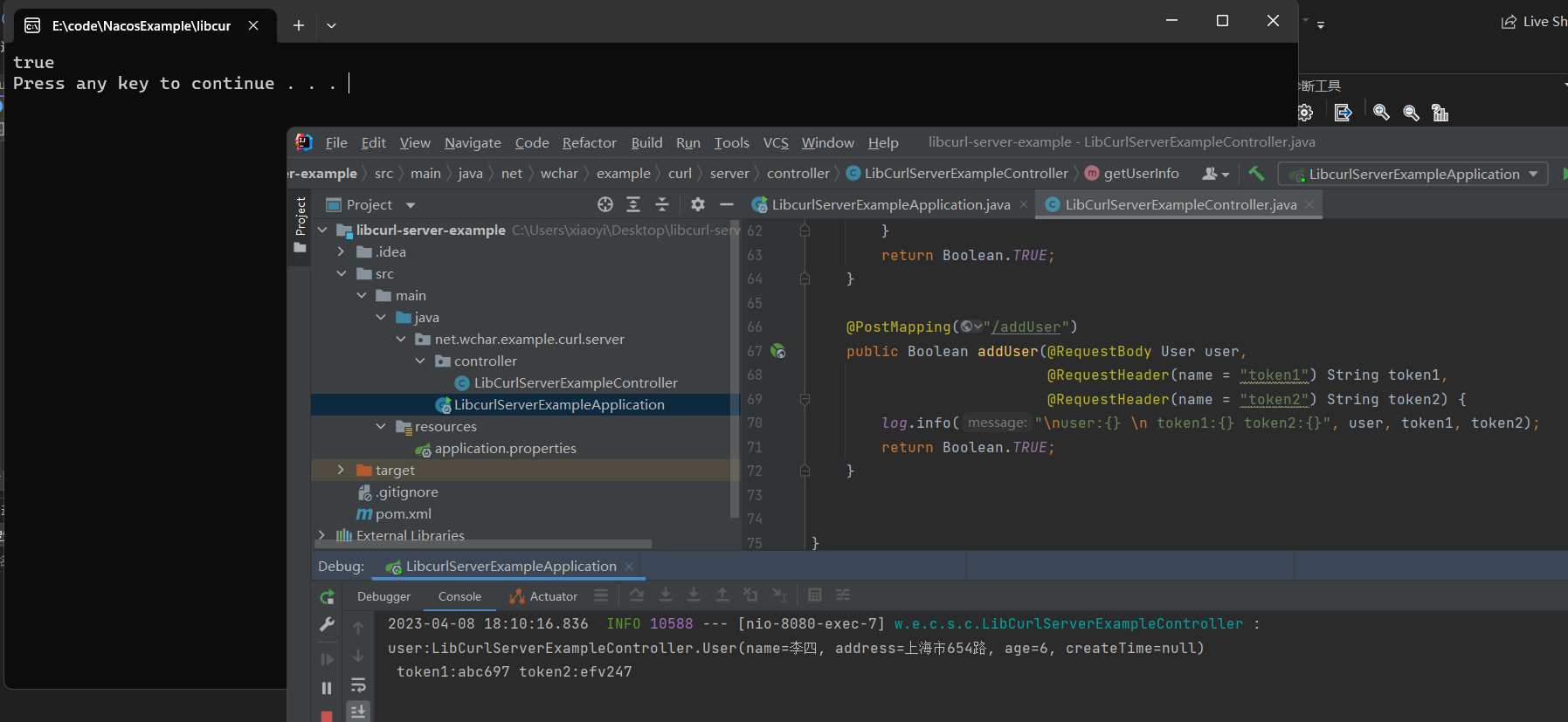
post form
服务端代码
1 | @PostMapping("/updateUser") |
c++ 代码
1 | /** |
测试调用
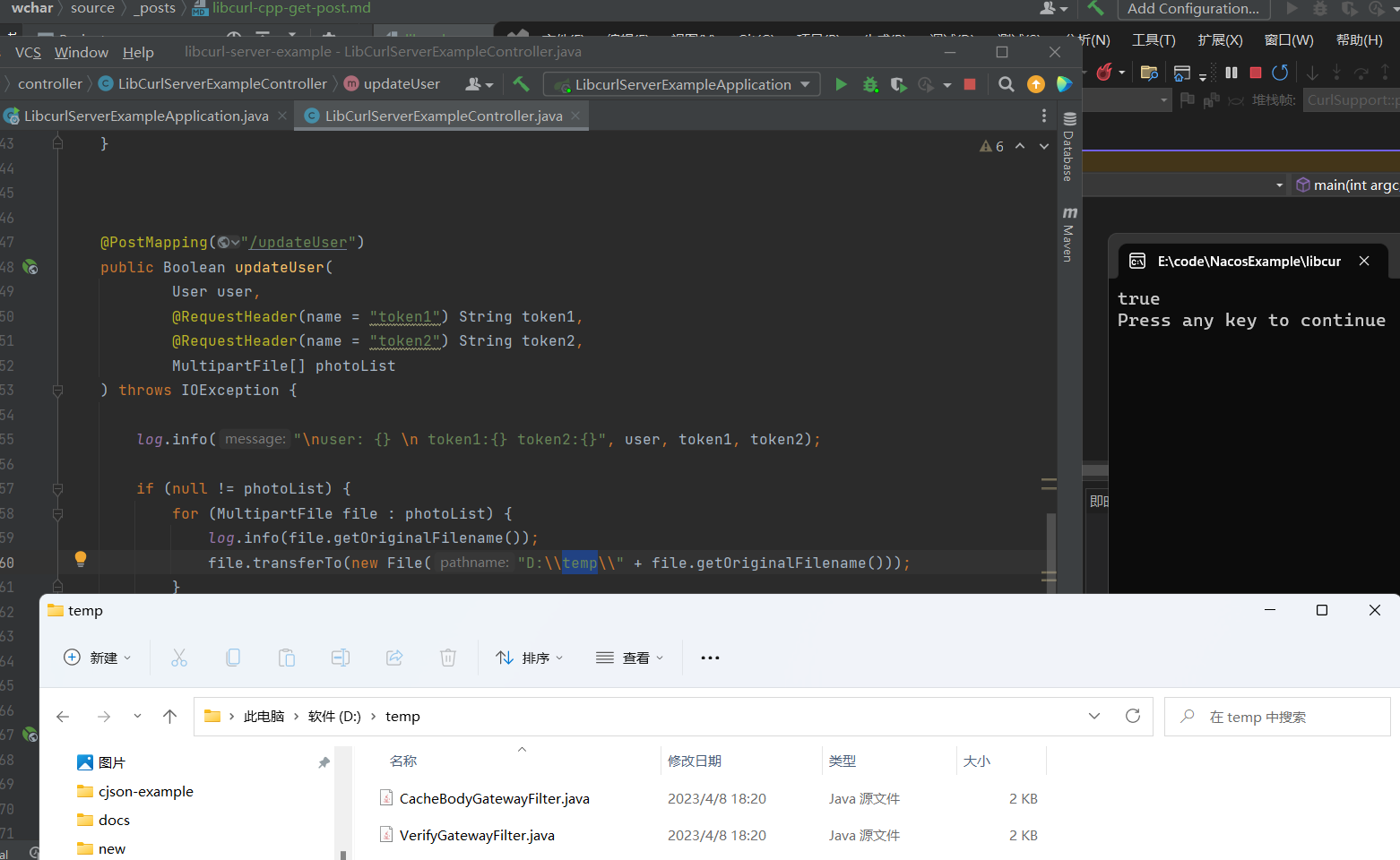
源代码
服务端代码:
https://github.com/wchar-net/libcurl-cpp-example-server
c++代码
https://github.com/wchar-net/libcurl-cpp-example
具体看源代码吧 o( ̄▽ ̄)ブ